13.3. Funktionsaufrufe#
Wenn Sie das Buch von vorne bis hierhin gelesen haben, werden Ihnen so einige Funktionsdefinitionen über den Weg gelaufen sein. Wie wir eigene Funktionen definieren, werden wir uns noch genauer ansehen. Vorerst begnügen wir uns mit dem Aufrufen von bereits existierenden Funktionen.
Vorab sei betont, dass eine mathematische Funktion sich von einer Python
-Funktion wesentlich unterscheidet.
Wir diskutieren dies genaue im Abschnitt Reinheit.
Wollen wir eine bestimmte Abfolge von Ausdrücken wieder und wieder aufrufen, so können wir diese Ausdrücke in einer Funktion kapseln. Diese Funktion erfüllt einen bestimmten Zweck.
Benutzten wir bereits existierende Funktionen, so kennen wir (jedenfalls sollte das so sein) das WAS aber nicht unbedingt das WIE. Das bedeutet für geübte Programmierer*innen, dass diese sich viel mit Dokumentationen diverser Module beschäftigen. Einerseits um das WAS in Erfahrung zu bringen und andererseits um eine Vorstellung vom WIE zu erhalten.
Wir rufen eine Funktion mit dem Namen name
auf, indem wir an den Namen runde Klammern anfügen: name(par1, par2)
.
Funktionen erwarten eine bestimmte Anzahl an Parametern (hier par1
, par2
), wobei es manchmal auch optionale Parameter geben kann.
Parameter und Argumente
Die in der Funktionsdefinition angegebenen Namen (Variablen) heißen Parameter. Die Werte, welche diese Parameter erhalten bezeichnen wir als Argumente des Funktionsaufrufs.
Argumente sind Werte, welche die Funktion erwartet und die wir übergeben müssen. Diese Argumente werden von der Funktion verarbeitet.
Zum Beispiel, bietet Python
die vordefinierte Funktion abs
an, welche eine Zahl als Argument erwartet.
abs(-23)
23
In diesem Fall realisiert diese Python
-Funktion eine mathematische Funktion, nämlich den Betrag \(\text{abs}(x) = |x|\).
Weitere Beispiele sind die Python
-Funktionen round
und max
.
round(5 - 1.3)
4
max(2, 3, 5 - 3, 3 * 3)
9
max(2, -10)
2
round
erwartet ebenfalls eine Zahl als Argument.
Die Funktion berechnet aus dem Argument die am nächsten liegende ganze Zahl und liefert diese zurück.
In anderen Worten, round
rundet zur nächst liegenden ganzen Zahl.
max
erwartet zwei oder mehr Zahlen als Argumente und liefert die größere Zahl zurück.
Bemerkenswert ist beim obigen Funktionsaufruf, dass die Ausdrücke 5-3
und 3 * 3
ausgewertet werden bevor die Funktion aufgerufen wird.
Das heißt, die Funktion wird mit den Argumenten 2, 3, 2
und 9
aufgerufen.
Wir sehen auch, dass max
mit einer unterschiedlichen Anzahl an Argumenten umgehen kann.
13.3.1. Module#
Es gibt nur wenige vordefinierte Funktionen, die keine Argumente besitzen.
Ein Beispiel hierfür ist die Funktion random()
des Moduls random
.
Module
Ein Python
-Modul ist eine Ansammlung von Funktionalität.
Es ist eine Zusammenstellung von Funktionen, welche zusammengehören.
Im Modul random
befinden sich, zum Beispiel, viele Funktionen die Operatoren aus der Wahrscheinlichkeitstheorie realisieren.
Um ein Modul nutzten zu können muss es, d.h. dessen Quellcode, auf Ihrem System installiert sein.
Manche Module, wie beispielsweise random
, gehören zur Standardbibliothek von Python
und werden mit Python
selbst installiert.
Ist das Modul installiert, müssen wir es in unseren Code importieren.
Wir machen es unserem Code bekannt, sodass wir es auch nutzten können.
Dies geschieht mit dem Schlüsselwort import
.
import random
random.random()
0.2521525738326792
Mit
import random
machen wir das Modul random
unter dem Namen random
bekannt.
In der zweiten Zeile des Codes rufen wir die Funktion random
des Moduls random
auf.
Diese kann ohne Argumente aufgerufen werden.
Wir können dem Modul auch einen anderen Namen verpassen. Mit
import random as rnd
machen wir das Modul random
unter dem Namen rnd
bekannt.
import random as rnd
rnd.random()
0.47397320724265546
Was macht diese Funktion? Wenn Sie die obige Zelle mehrfach ausführen werden Sie feststellen, dass sie uns eine zufällige Fließkommazahl zwischen 0 und 1 zurückliefert.
Funktionen, Module und auch Funktionen von Modulen enthalten oft eine Dokumentation in Form von Kommentaren.
Wir können uns deshalb Informationen zu dem WAS (und manchmal auch zu dem WIE) einer Funktion holen.
Hierzu schreiben wir den Funktionsnamen ohne die runden Klammern und fügen ein ?
an.
Alternativ können Sie auch die Hilfefunktion help
verwenden: help(random.random)
.
import random
help(random.random)
Help on built-in function random:
random() method of random.Random instance
random() -> x in the interval [0, 1).
Die Ausgabe lautet:
Help on built-in function random:
random() method of random.Random instance
random() -> x in the interval [0, 1).
random() -> x
bedeutet, dass random()
den Wert x
zurückgibt, wobei x
im halb offenen Intervall \([0;1)\) liegt.
In anderen Worten: Die Funktion random()
liefert einen Wert zwischen 0 und 1 zurück, wobei die 1 ausgeschlossen ist.
Exercise 13.1 (Hilfe)
Nutzten Sie die eingebaute Hilfe und betrachten Sie die Ausgabe von help(max)
bzw. max?
.
Beschreiben Sie die möglichen Argumente der Funktion max
und das WAS jener Funktion.
Sie müssen nicht jedes Wort verstehen aber ziehen Sie ihre Schlüsse.
Solution to Exercise 13.1 (Hilfe)
Neben der optionalen Argumente, erwartet max
entweder ein iterierbares Argument oder aber mindestens zwei Argumente.
Im ersten Fall gibt max
das größte Element des iterierbaren Arguments zurück.
D.h., ein iterierbares Argument ist wohl so etwas wie eine Sequenz, Liste, Menge an Elementen.
13.3.2. Auswertungsreihenfolge#
Ein Funktionsaufruf ist ebenfalls ein Ausdruck und, wie beim Rechnen, werden Ausdrücke von innen nach außen ausgewertet. Blicken Sie auf folgenden Ausdruck:
round(max(abs(-abs(-3)), 3, 5 + 3) - 0.6)
7
Dieser Ausdruck besteht aus mehreren Ausdrücken. Die Auswertung des gesamten Ausdrucks erfolgt von links nach rechts, wobei die notwendigen Argumente, welche durch einen Ausdruck berechnet werden, ausgewertet werden. Deshalb springen wir von außen nach innen und werten dann von innen nach außen aus.
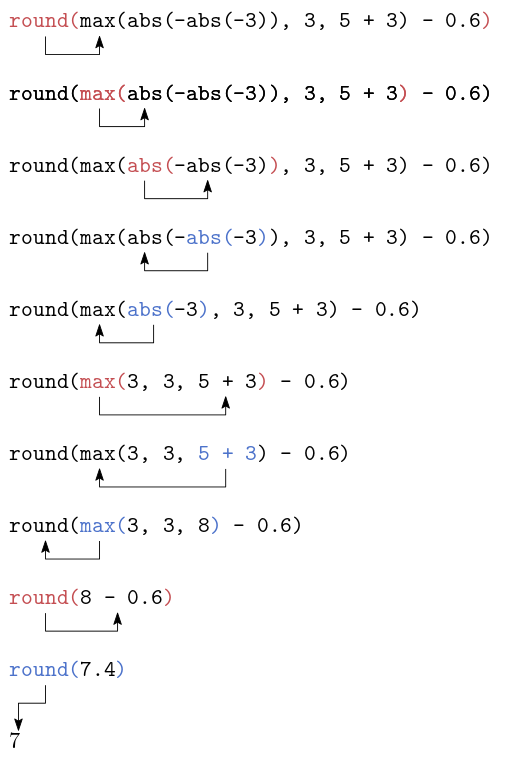
Abb. 13.5 Skizze der Auswertung / Evaluierung des obigen Ausdrucks.#
Um round
auszuwerten muss zu aller erst der Ausdruck max(abs(-abs(-3)), 3, 5 + 3) - 0.6
ausgewertet werden.
Deshalb wird max(abs(-abs(-3)), 3, 5 + 3)
ausgewertet.
Um jedoch max
auszuwerten werden alle Argumente von links nach rechts berechnet.
Es beginnt mit dem ersten Argument: abs(-abs(-3))
wird zu abs(-3)
wird zu 3
und wir erhalten max(3, 3, 5 + 3)
.
Das zweite Argument ist bereits ausgewertet und aus 5+3
wird 8
.
Schlussendlich wird max(3, 3, 8)
zu 8
.
Was bleibt ist round(8 - 0.6)
was zu round(7.4)
ausgewertet wird.
Dieser Ausdruck ergibt 7
.
Wir können jeder Variablen auch einen Ausdruck zuweisen. Dieser wird ausgewertet und das Ergebnis wird der Variablen zugewiesen.
x = round(max(abs(-abs(-3)), 3, 5 + 3) - 0.6)
x
7
Auch können wir Funktionsausdrücke mit anderen Ausdrucken wie etwa arithmetische Operatoren kombinieren. Durch die folgende Abfolge von Ausdrücken berechnen wir den prozentualen Anteil der Einwohner in Deutschland, die in München wohnen und zwar auf zwei Nachkommastellen gerundet:
population_munich = 1_553_373
population_germany = 83_121_363
persentage = round(10000 * 1_553_373 / 83_121_363) / 100
persentage
1.87
13.3.3. Das Kennenlernen#
Programmieren beginnt oft mit dem Durchwühlen von Dokumentationen fremder Module und Pakete. Bevor wir loslegen müssen wir erst in Erfahrung bringen WAS überhaupt möglich ist. Welche vordefinierten Funktionen und welche Module gibt es bzw. welche dieser Module könnten für meine Zwecke nützlich sein.
Selbst nach Jahren an Programmierkenntnissen hört dieser Lernprozess nie auf. Ständig werden neue nützliche Module programmiert und auch Sie werden noch irgendwann Ihre eigenen Module implementieren und nutzten. Einer der wichtigsten Fähigkeiten ist es, Dokumentationen zu finden und richtig zu lesen.
Mit zunehmender Erfahrung klappt auch dies immer besser.
Zum Beispiel würden erfahrene Programmierer*innen richtigerweise vermuten, dass das Modul random
auch eine Funktion bieten wird, welche eine zufällige natürliche Zahl zurückliefert.
Durchforsten wir das Internet nach diesem Modul so stoßen wir möglicherweise auf diese Seite.
Dort finden wir eine Funktion random.randint
.
Wir finden diese auch in der Hilfe, die wir duch help
ausgeben können.
import random
help(random)
Help on module random:
NAME
random - Random variable generators.
MODULE REFERENCE
https://docs.python.org/3.11/library/random.html
The following documentation is automatically generated from the Python
source files. It may be incomplete, incorrect or include features that
are considered implementation detail and may vary between Python
implementations. When in doubt, consult the module reference at the
location listed above.
DESCRIPTION
bytes
-----
uniform bytes (values between 0 and 255)
integers
--------
uniform within range
sequences
---------
pick random element
pick random sample
pick weighted random sample
generate random permutation
distributions on the real line:
------------------------------
uniform
triangular
normal (Gaussian)
lognormal
negative exponential
gamma
beta
pareto
Weibull
distributions on the circle (angles 0 to 2pi)
---------------------------------------------
circular uniform
von Mises
General notes on the underlying Mersenne Twister core generator:
* The period is 2**19937-1.
* It is one of the most extensively tested generators in existence.
* The random() method is implemented in C, executes in a single Python step,
and is, therefore, threadsafe.
CLASSES
_random.Random(builtins.object)
Random
SystemRandom
class Random(_random.Random)
| Random(x=None)
|
| Random number generator base class used by bound module functions.
|
| Used to instantiate instances of Random to get generators that don't
| share state.
|
| Class Random can also be subclassed if you want to use a different basic
| generator of your own devising: in that case, override the following
| methods: random(), seed(), getstate(), and setstate().
| Optionally, implement a getrandbits() method so that randrange()
| can cover arbitrarily large ranges.
|
| Method resolution order:
| Random
| _random.Random
| builtins.object
|
| Methods defined here:
|
| __getstate__(self)
| Helper for pickle.
|
| __init__(self, x=None)
| Initialize an instance.
|
| Optional argument x controls seeding, as for Random.seed().
|
| __reduce__(self)
| Helper for pickle.
|
| __setstate__(self, state)
|
| betavariate(self, alpha, beta)
| Beta distribution.
|
| Conditions on the parameters are alpha > 0 and beta > 0.
| Returned values range between 0 and 1.
|
| choice(self, seq)
| Choose a random element from a non-empty sequence.
|
| choices(self, population, weights=None, *, cum_weights=None, k=1)
| Return a k sized list of population elements chosen with replacement.
|
| If the relative weights or cumulative weights are not specified,
| the selections are made with equal probability.
|
| expovariate(self, lambd)
| Exponential distribution.
|
| lambd is 1.0 divided by the desired mean. It should be
| nonzero. (The parameter would be called "lambda", but that is
| a reserved word in Python.) Returned values range from 0 to
| positive infinity if lambd is positive, and from negative
| infinity to 0 if lambd is negative.
|
| gammavariate(self, alpha, beta)
| Gamma distribution. Not the gamma function!
|
| Conditions on the parameters are alpha > 0 and beta > 0.
|
| The probability distribution function is:
|
| x ** (alpha - 1) * math.exp(-x / beta)
| pdf(x) = --------------------------------------
| math.gamma(alpha) * beta ** alpha
|
| gauss(self, mu=0.0, sigma=1.0)
| Gaussian distribution.
|
| mu is the mean, and sigma is the standard deviation. This is
| slightly faster than the normalvariate() function.
|
| Not thread-safe without a lock around calls.
|
| getstate(self)
| Return internal state; can be passed to setstate() later.
|
| lognormvariate(self, mu, sigma)
| Log normal distribution.
|
| If you take the natural logarithm of this distribution, you'll get a
| normal distribution with mean mu and standard deviation sigma.
| mu can have any value, and sigma must be greater than zero.
|
| normalvariate(self, mu=0.0, sigma=1.0)
| Normal distribution.
|
| mu is the mean, and sigma is the standard deviation.
|
| paretovariate(self, alpha)
| Pareto distribution. alpha is the shape parameter.
|
| randbytes(self, n)
| Generate n random bytes.
|
| randint(self, a, b)
| Return random integer in range [a, b], including both end points.
|
| randrange(self, start, stop=None, step=1)
| Choose a random item from range(stop) or range(start, stop[, step]).
|
| Roughly equivalent to ``choice(range(start, stop, step))`` but
| supports arbitrarily large ranges and is optimized for common cases.
|
| sample(self, population, k, *, counts=None)
| Chooses k unique random elements from a population sequence.
|
| Returns a new list containing elements from the population while
| leaving the original population unchanged. The resulting list is
| in selection order so that all sub-slices will also be valid random
| samples. This allows raffle winners (the sample) to be partitioned
| into grand prize and second place winners (the subslices).
|
| Members of the population need not be hashable or unique. If the
| population contains repeats, then each occurrence is a possible
| selection in the sample.
|
| Repeated elements can be specified one at a time or with the optional
| counts parameter. For example:
|
| sample(['red', 'blue'], counts=[4, 2], k=5)
|
| is equivalent to:
|
| sample(['red', 'red', 'red', 'red', 'blue', 'blue'], k=5)
|
| To choose a sample from a range of integers, use range() for the
| population argument. This is especially fast and space efficient
| for sampling from a large population:
|
| sample(range(10000000), 60)
|
| seed(self, a=None, version=2)
| Initialize internal state from a seed.
|
| The only supported seed types are None, int, float,
| str, bytes, and bytearray.
|
| None or no argument seeds from current time or from an operating
| system specific randomness source if available.
|
| If *a* is an int, all bits are used.
|
| For version 2 (the default), all of the bits are used if *a* is a str,
| bytes, or bytearray. For version 1 (provided for reproducing random
| sequences from older versions of Python), the algorithm for str and
| bytes generates a narrower range of seeds.
|
| setstate(self, state)
| Restore internal state from object returned by getstate().
|
| shuffle(self, x)
| Shuffle list x in place, and return None.
|
| triangular(self, low=0.0, high=1.0, mode=None)
| Triangular distribution.
|
| Continuous distribution bounded by given lower and upper limits,
| and having a given mode value in-between.
|
| http://en.wikipedia.org/wiki/Triangular_distribution
|
| uniform(self, a, b)
| Get a random number in the range [a, b) or [a, b] depending on rounding.
|
| vonmisesvariate(self, mu, kappa)
| Circular data distribution.
|
| mu is the mean angle, expressed in radians between 0 and 2*pi, and
| kappa is the concentration parameter, which must be greater than or
| equal to zero. If kappa is equal to zero, this distribution reduces
| to a uniform random angle over the range 0 to 2*pi.
|
| weibullvariate(self, alpha, beta)
| Weibull distribution.
|
| alpha is the scale parameter and beta is the shape parameter.
|
| ----------------------------------------------------------------------
| Class methods defined here:
|
| __init_subclass__(**kwargs) from builtins.type
| Control how subclasses generate random integers.
|
| The algorithm a subclass can use depends on the random() and/or
| getrandbits() implementation available to it and determines
| whether it can generate random integers from arbitrarily large
| ranges.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| VERSION = 3
|
| ----------------------------------------------------------------------
| Methods inherited from _random.Random:
|
| getrandbits(self, k, /)
| getrandbits(k) -> x. Generates an int with k random bits.
|
| random(self, /)
| random() -> x in the interval [0, 1).
|
| ----------------------------------------------------------------------
| Static methods inherited from _random.Random:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
class SystemRandom(Random)
| SystemRandom(x=None)
|
| Alternate random number generator using sources provided
| by the operating system (such as /dev/urandom on Unix or
| CryptGenRandom on Windows).
|
| Not available on all systems (see os.urandom() for details).
|
| Method resolution order:
| SystemRandom
| Random
| _random.Random
| builtins.object
|
| Methods defined here:
|
| getrandbits(self, k)
| getrandbits(k) -> x. Generates an int with k random bits.
|
| getstate = _notimplemented(self, *args, **kwds)
|
| randbytes(self, n)
| Generate n random bytes.
|
| random(self)
| Get the next random number in the range 0.0 <= X < 1.0.
|
| seed(self, *args, **kwds)
| Stub method. Not used for a system random number generator.
|
| setstate = _notimplemented(self, *args, **kwds)
|
| ----------------------------------------------------------------------
| Methods inherited from Random:
|
| __getstate__(self)
| Helper for pickle.
|
| __init__(self, x=None)
| Initialize an instance.
|
| Optional argument x controls seeding, as for Random.seed().
|
| __reduce__(self)
| Helper for pickle.
|
| __setstate__(self, state)
|
| betavariate(self, alpha, beta)
| Beta distribution.
|
| Conditions on the parameters are alpha > 0 and beta > 0.
| Returned values range between 0 and 1.
|
| choice(self, seq)
| Choose a random element from a non-empty sequence.
|
| choices(self, population, weights=None, *, cum_weights=None, k=1)
| Return a k sized list of population elements chosen with replacement.
|
| If the relative weights or cumulative weights are not specified,
| the selections are made with equal probability.
|
| expovariate(self, lambd)
| Exponential distribution.
|
| lambd is 1.0 divided by the desired mean. It should be
| nonzero. (The parameter would be called "lambda", but that is
| a reserved word in Python.) Returned values range from 0 to
| positive infinity if lambd is positive, and from negative
| infinity to 0 if lambd is negative.
|
| gammavariate(self, alpha, beta)
| Gamma distribution. Not the gamma function!
|
| Conditions on the parameters are alpha > 0 and beta > 0.
|
| The probability distribution function is:
|
| x ** (alpha - 1) * math.exp(-x / beta)
| pdf(x) = --------------------------------------
| math.gamma(alpha) * beta ** alpha
|
| gauss(self, mu=0.0, sigma=1.0)
| Gaussian distribution.
|
| mu is the mean, and sigma is the standard deviation. This is
| slightly faster than the normalvariate() function.
|
| Not thread-safe without a lock around calls.
|
| lognormvariate(self, mu, sigma)
| Log normal distribution.
|
| If you take the natural logarithm of this distribution, you'll get a
| normal distribution with mean mu and standard deviation sigma.
| mu can have any value, and sigma must be greater than zero.
|
| normalvariate(self, mu=0.0, sigma=1.0)
| Normal distribution.
|
| mu is the mean, and sigma is the standard deviation.
|
| paretovariate(self, alpha)
| Pareto distribution. alpha is the shape parameter.
|
| randint(self, a, b)
| Return random integer in range [a, b], including both end points.
|
| randrange(self, start, stop=None, step=1)
| Choose a random item from range(stop) or range(start, stop[, step]).
|
| Roughly equivalent to ``choice(range(start, stop, step))`` but
| supports arbitrarily large ranges and is optimized for common cases.
|
| sample(self, population, k, *, counts=None)
| Chooses k unique random elements from a population sequence.
|
| Returns a new list containing elements from the population while
| leaving the original population unchanged. The resulting list is
| in selection order so that all sub-slices will also be valid random
| samples. This allows raffle winners (the sample) to be partitioned
| into grand prize and second place winners (the subslices).
|
| Members of the population need not be hashable or unique. If the
| population contains repeats, then each occurrence is a possible
| selection in the sample.
|
| Repeated elements can be specified one at a time or with the optional
| counts parameter. For example:
|
| sample(['red', 'blue'], counts=[4, 2], k=5)
|
| is equivalent to:
|
| sample(['red', 'red', 'red', 'red', 'blue', 'blue'], k=5)
|
| To choose a sample from a range of integers, use range() for the
| population argument. This is especially fast and space efficient
| for sampling from a large population:
|
| sample(range(10000000), 60)
|
| shuffle(self, x)
| Shuffle list x in place, and return None.
|
| triangular(self, low=0.0, high=1.0, mode=None)
| Triangular distribution.
|
| Continuous distribution bounded by given lower and upper limits,
| and having a given mode value in-between.
|
| http://en.wikipedia.org/wiki/Triangular_distribution
|
| uniform(self, a, b)
| Get a random number in the range [a, b) or [a, b] depending on rounding.
|
| vonmisesvariate(self, mu, kappa)
| Circular data distribution.
|
| mu is the mean angle, expressed in radians between 0 and 2*pi, and
| kappa is the concentration parameter, which must be greater than or
| equal to zero. If kappa is equal to zero, this distribution reduces
| to a uniform random angle over the range 0 to 2*pi.
|
| weibullvariate(self, alpha, beta)
| Weibull distribution.
|
| alpha is the scale parameter and beta is the shape parameter.
|
| ----------------------------------------------------------------------
| Class methods inherited from Random:
|
| __init_subclass__(**kwargs) from builtins.type
| Control how subclasses generate random integers.
|
| The algorithm a subclass can use depends on the random() and/or
| getrandbits() implementation available to it and determines
| whether it can generate random integers from arbitrarily large
| ranges.
|
| ----------------------------------------------------------------------
| Data descriptors inherited from Random:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
|
| ----------------------------------------------------------------------
| Data and other attributes inherited from Random:
|
| VERSION = 3
|
| ----------------------------------------------------------------------
| Static methods inherited from _random.Random:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
FUNCTIONS
betavariate(alpha, beta) method of Random instance
Beta distribution.
Conditions on the parameters are alpha > 0 and beta > 0.
Returned values range between 0 and 1.
choice(seq) method of Random instance
Choose a random element from a non-empty sequence.
choices(population, weights=None, *, cum_weights=None, k=1) method of Random instance
Return a k sized list of population elements chosen with replacement.
If the relative weights or cumulative weights are not specified,
the selections are made with equal probability.
expovariate(lambd) method of Random instance
Exponential distribution.
lambd is 1.0 divided by the desired mean. It should be
nonzero. (The parameter would be called "lambda", but that is
a reserved word in Python.) Returned values range from 0 to
positive infinity if lambd is positive, and from negative
infinity to 0 if lambd is negative.
gammavariate(alpha, beta) method of Random instance
Gamma distribution. Not the gamma function!
Conditions on the parameters are alpha > 0 and beta > 0.
The probability distribution function is:
x ** (alpha - 1) * math.exp(-x / beta)
pdf(x) = --------------------------------------
math.gamma(alpha) * beta ** alpha
gauss(mu=0.0, sigma=1.0) method of Random instance
Gaussian distribution.
mu is the mean, and sigma is the standard deviation. This is
slightly faster than the normalvariate() function.
Not thread-safe without a lock around calls.
getrandbits(k, /) method of Random instance
getrandbits(k) -> x. Generates an int with k random bits.
getstate() method of Random instance
Return internal state; can be passed to setstate() later.
lognormvariate(mu, sigma) method of Random instance
Log normal distribution.
If you take the natural logarithm of this distribution, you'll get a
normal distribution with mean mu and standard deviation sigma.
mu can have any value, and sigma must be greater than zero.
normalvariate(mu=0.0, sigma=1.0) method of Random instance
Normal distribution.
mu is the mean, and sigma is the standard deviation.
paretovariate(alpha) method of Random instance
Pareto distribution. alpha is the shape parameter.
randbytes(n) method of Random instance
Generate n random bytes.
randint(a, b) method of Random instance
Return random integer in range [a, b], including both end points.
random() method of Random instance
random() -> x in the interval [0, 1).
randrange(start, stop=None, step=1) method of Random instance
Choose a random item from range(stop) or range(start, stop[, step]).
Roughly equivalent to ``choice(range(start, stop, step))`` but
supports arbitrarily large ranges and is optimized for common cases.
sample(population, k, *, counts=None) method of Random instance
Chooses k unique random elements from a population sequence.
Returns a new list containing elements from the population while
leaving the original population unchanged. The resulting list is
in selection order so that all sub-slices will also be valid random
samples. This allows raffle winners (the sample) to be partitioned
into grand prize and second place winners (the subslices).
Members of the population need not be hashable or unique. If the
population contains repeats, then each occurrence is a possible
selection in the sample.
Repeated elements can be specified one at a time or with the optional
counts parameter. For example:
sample(['red', 'blue'], counts=[4, 2], k=5)
is equivalent to:
sample(['red', 'red', 'red', 'red', 'blue', 'blue'], k=5)
To choose a sample from a range of integers, use range() for the
population argument. This is especially fast and space efficient
for sampling from a large population:
sample(range(10000000), 60)
seed(a=None, version=2) method of Random instance
Initialize internal state from a seed.
The only supported seed types are None, int, float,
str, bytes, and bytearray.
None or no argument seeds from current time or from an operating
system specific randomness source if available.
If *a* is an int, all bits are used.
For version 2 (the default), all of the bits are used if *a* is a str,
bytes, or bytearray. For version 1 (provided for reproducing random
sequences from older versions of Python), the algorithm for str and
bytes generates a narrower range of seeds.
setstate(state) method of Random instance
Restore internal state from object returned by getstate().
shuffle(x) method of Random instance
Shuffle list x in place, and return None.
triangular(low=0.0, high=1.0, mode=None) method of Random instance
Triangular distribution.
Continuous distribution bounded by given lower and upper limits,
and having a given mode value in-between.
http://en.wikipedia.org/wiki/Triangular_distribution
uniform(a, b) method of Random instance
Get a random number in the range [a, b) or [a, b] depending on rounding.
vonmisesvariate(mu, kappa) method of Random instance
Circular data distribution.
mu is the mean angle, expressed in radians between 0 and 2*pi, and
kappa is the concentration parameter, which must be greater than or
equal to zero. If kappa is equal to zero, this distribution reduces
to a uniform random angle over the range 0 to 2*pi.
weibullvariate(alpha, beta) method of Random instance
Weibull distribution.
alpha is the scale parameter and beta is the shape parameter.
DATA
__all__ = ['Random', 'SystemRandom', 'betavariate', 'choice', 'choices...
FILE
/opt/homebrew/Caskroom/miniconda/base/envs/ct-book/lib/python3.11/random.py
Exercise 13.2 (Dokumentation)
Finden Sie die Dokumentation der Funktion random.shuffle
und beschreiben Sie anhand der Dokumentation das WAS dieser Funktion.
Solution to Exercise 13.2 (Dokumentation)
Diese Funktion mischt eine gegebene Sequenz.
Die übergebene Sequenz x
wird dadurch verändert (durchgemischt).